Joomla Select Options with Style
add Style and Attributes to Joomla Select Options.
A brief tutorial to show how to style individual options within a select.genericlist.
The Joomla html library includes the class select which can be used to generate groups of radio or combo buttons and select boxes.
With increasing ajax requirements it is often necessary to perform some logic on the client forms.
When selects come into play, we often have to resort to ajax calls to update some controls depending on which option is selected.
Adding some extra params to the options allows to create easier interactive code that manipulates them with a single jQuery('option.myclass'), in combination with some css.
To create a select box we use the function:
PHP Code:JHTML::_('select.genericlist',...);
which invokes the method genericlist() in /libraries/joomla/html/html/select.php
and we have two ways of providing the structure which holds the options:
a) create an array of objects each having, at least, two properties: value and text;
b) create individual options with a call to JHTML:: _('select.option',...);
While the first approach is very handy when creating a lookup list straight from the database:
PHP Code:
$db->setQuery('SELECT alias AS value, title AS text FROM #__content '); $arts = $db->loadObjectList(); echo JHTML::_('select.genericlist','','article',array('class'=>'arts');
the second is by far more common:
PHP Code:
$listOptions[] = JHTML::_('select.option', '1', 'some text n.1' ); echo JHTML::_('select.genericlist', $listingTypesOptions, 'article', …
Get to the point. How do I style the options?
Depending on your situation (case a. or case b.) you need to follow a different approach.
The solution to case a. was presented http://stackoverflow.com/questions/10918584/adding-attributes-to-select-options-in-joomla.
Regarding case b., I wasn't able to find a satisfactory (i.e. Working) answer anywhere, so I gathered some tips here http://joomla-support.ru/archive/index.php/t-29437.html and looking at the source code of select.php. Without further ado, the code:
This comes from a real project, loading the listing types of the joomlistings component.
PHP Code:
/** * Builds the select and options for the listing types. * the listing types are styled based on their aliases for usage by jQuery in the * gshare module's view and adcustom's view post. * * @param unknown_type $selectName * @param unknown_type $listingTypes the array coming from a db query - loadObjectList which holds the values of the listing types. * @param unknown_type $selectedValue the (pre)selected value * @param unknown_type $tooltip the tooltip for the select; * @param unknown_type $extraClass an extra css class (or classes) to be added to the select * @param unknown_type $translatePrefix the alias will be prefixed by this string before attempting to translate it * @param unknown_type $createEmpty should I create an empty option at the very top? this would be its text. * @return Ambigous <mixed, boolean> */ function getListingTypesHTML($selectName,$listingTypes,$selectedValue,$tooltip,$extraClass,$createEmpty=false) { $listsHTMLAttrs = array('title'=>$tooltip, 'class'=>$extraClass); /* the options.attr is tricky: it needs to be a string token and it must be identical * for each option and the select attributes. Here I chose extraOptionAttributes, * all that's required is that the two match. */ $listsAttrs = array('id'=>$selectName,'list.attr'=>$listsHTMLAttrs, 'list.select'=>$selectedValue, 'option.attr'=>'extraOptionAttributes'); $listingTypesOptions = array(); if ($createEmpty) { // style the first option control: $optionsHTMLAttrs = array('class'=>'emptyoption'); //, 'title'=>'option sample title'); $optionsAttrs = array('attr'=>$optionsHTMLAttrs, 'option.attr'=>'extraOptionAttributes'); $listingTypesOptions[] = JHTML::_('select.option', '', $createEmpty, $optionsAttrs ); } foreach ($listingTypes as $listingType) { /** * To make selections simpler in the jQuery manipulations, I need to * - preselect the right options based on tourist / residential current option * - add a meaningful class to options so I may add/remove top level classes and * automatically show/hide * the options (much like price_to extra-7 is toggled in the gshare.php view) */ $alias = $listingType->alias; if (strpos($alias,'search_')===0) { // only residential: $className = 'search residential'; } else { // both tourist and residential: $className = 'rent '; if (strpos($alias,'_bb') || strpos($alias,'_couch')) { $className .= 'tourist'; } else { $className .= 'residential'; } } $className .= " $alias"; $optionsHTMLAttrs = array('class'=>$className); $optionsAttrs = array('attr'=>$optionsHTMLAttrs, 'option.attr'=>'extraOptionAttributes'); $listingTypesOptions[] = JHTML::_('select.option', $listingType->alias, $listingType->alias, $optionsAttrs ); } return JHTML::_('select.genericlist', $listingTypesOptions, $selectName, $listsAttrs); }
Invoking this function
This function is invoked like this:
PHP Code:
$listingTypes=modJLGshareSearchHelper::getListingTypes(); $selectName = 'listingtype'; $listingTypesHTML = modJLGshareSearchHelper::getListingTypesHTML('listingtype',$listingTypes,JRequest::getVar('listingtype',''),JText::_('SEARCHBOX_LISTINGTYPE_DESC',''),'styled',JText::_('SEARCH_LISTING_TYPE'));
And the $listingTypes is the result of loadObjectList() from the database:
PHP Code:
/** * return a list of available Listing Types (see administrator>listings->listing types * @return Ambigous <mixed, NULL, multitype:unknown mixed > */ function getListingTypes() { $db=JFactory::getDBO(); $query="SELECT id, title, alias, description FROM #__jltypes WHERE published='1' ORDER BY ordering"; $db->setQuery($query); return $db->loadObjectList(); }
HTML representation:
This piece of code generates the following markup
HTML Code:
<select id="listingtype" class="styled emptyoption custom-hidden-select" style="width: 99px;" title="Choose the listing type you are searching" name="listingtype"><option class="emptyoption" selected="selected" value="">what...</option><option class="rent residential rent_room" value="rent_room">Room in shared house</option><option class="rent residential rent_house" value="rent_house">Whole apartment</option><option class="search residential search_room" value="search_room">People with whom I will share my house</option><option class="search residential search_house" value="search_house">Tenants for an empty house</option><option class="rent tourist rent_bb" value="rent_bb">Bed & breakfast</option><option class="rent tourist rent_couch" value="rent_couch">Couch Surfing</option> </select>
The result:
This is what I achieve with a simple jQuery script which toggles a class on the form container and a css which – based on the classes combination – shows or hides selected options:
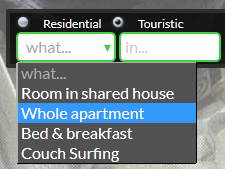
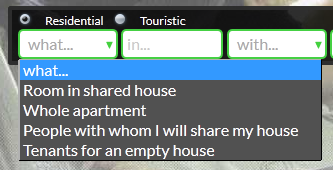
As you can see, some options are toggled (shown/hidden) when the radio button above are clicked.
The radio button is bound to a jQuery function which will toggle the class of the div containing the select:
Javascript Code:
jQuery("div.topsearchbuttons input").change(function(obj) { isTourist = (jQuery("div.topsearchbuttons input[name='srctourist']:checked").val()=='tourist'); if (isTourist) { jQuery("div#searchconditions").addClass('style-tourist').removeClass('style-residential'); } else { jQuery("div#searchconditions").removeClass('style-tourist').addClass('style-residential'); } });
The css does the rest:
CSS Code:div#searchconditions.style-residential select#listingtype option.tourist {display:none}
See it in action
Live on www.gay-houseshare.com
A word of caution
While this approach is effective, the way different browsers display styled options varies, so you should use a different type of control to ensure proper display. Even at the end of 2012 most browsers don't support transparent colors (rgba) as background of options.
If you choose to use a select box, which is excellent for accessibility and compatibility, there are neat scripts that will replace the select with different components, often inheriting the classes and behaviour (click etc.) of the original selects and options, making these approaches very compatible with the code presented. Some of these tools include jquery.ui.cutom.select
http://www.georgepaterson.com/sandbox/jquery-ui-custom-select-demo/
and selectBox:
http://labs.abeautifulsite.net/jquery-selectBox/